TestNG – Dependency Test
In TestNG, we use dependOnMethods
and dependsOnGroups
to implement dependency testing. If a dependent method is fail, all the subsequent test methods will be skipped, NOT failed.
1. dependOnMethods Example
A simple example, “method2()” is dependent on “method1()”.
1.1 If method1()
is passed, method2()
will be executed.
App.java
package com.mkyong.testng.examples.dependency;
import org.testng.annotations.Test;
public class App {
@Test
public void method1() {
System.out.println("This is method 1");
}
@Test(dependsOnMethods = { "method1" })
public void method2() {
System.out.println("This is method 2");
}
}
Output
This is method 1
This is method 2
PASSED: method1
PASSED: method2
===============================================
Default test
Tests run: 2, Failures: 0, Skips: 0
===============================================
1.2 If method1()
is failed, method2()
will be skipped.
App.java
package com.mkyong.testng.examples.dependency;
import org.testng.annotations.Test;
public class App {
//This test will be failed.
@Test
public void method1() {
System.out.println("This is method 1");
throw new RuntimeException();
}
@Test(dependsOnMethods = { "method1" })
public void method2() {
System.out.println("This is method 2");
}
}
Output
This is method 1
FAILED: method1
java.lang.RuntimeException
at com.mkyong.testng.examples.dependency.App.method1(App.java:10)
//...
SKIPPED: method2
===============================================
Default test
Tests run: 2, Failures: 1, Skips: 1
===============================================
2. dependsOnGroups Example
Let create few test cases to demonstrate the mixed use of dependsOnMethods
and dependsOnGroups
. See comments for self-explanatory.
TestServer.java
package com.mkyong.testng.examples.dependency;
import org.testng.annotations.Test;
//all methods of this class are belong to "deploy" group.
@Test(groups="deploy")
public class TestServer {
@Test
public void deployServer() {
System.out.println("Deploying Server...");
}
//Run this if deployServer() is passed.
@Test(dependsOnMethods="deployServer")
public void deployBackUpServer() {
System.out.println("Deploying Backup Server...");
}
}
TestDatabase.java
package com.mkyong.testng.examples.dependency;
import org.testng.annotations.Test;
public class TestDatabase {
//belong to "db" group,
//Run if all methods from "deploy" group are passed.
@Test(groups="db", dependsOnGroups="deploy")
public void initDB() {
System.out.println("This is initDB()");
}
//belong to "db" group,
//Run if "initDB" method is passed.
@Test(dependsOnMethods = { "initDB" }, groups="db")
public void testConnection() {
System.out.println("This is testConnection()");
}
}
TestApp.java
package com.mkyong.testng.examples.dependency;
import org.testng.annotations.Test;
public class TestApp {
//Run if all methods from "deploy" and "db" groups are passed.
@Test(dependsOnGroups={"deploy","db"})
public void method1() {
System.out.println("This is method 1");
//throw new RuntimeException();
}
//Run if method1() is passed.
@Test(dependsOnMethods = { "method1" })
public void method2() {
System.out.println("This is method 2");
}
}
Create a XML file and test them together.
testng.xml
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="TestDependency">
<test name="TestCase1">
<classes>
<class
name="com.mkyong.testng.examples.dependency.TestApp">
</class>
<class
name="com.mkyong.testng.examples.dependency.TestDatabase">
</class>
<class
name="com.mkyong.testng.examples.dependency.TestServer">
</class>
</classes>
</test>
</suite>
Output
Deploying Server...
Deploying Backup Server...
This is initDB()
This is testConnection()
This is method 1
This is method 2
===============================================
TestDependency
Total tests run: 6, Failures: 0, Skips: 0
===============================================
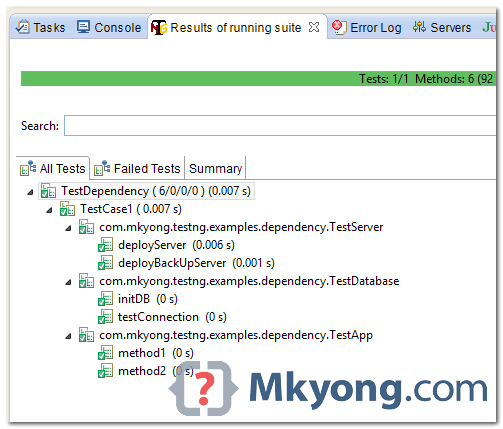
Hi,
i have tried the same as demonstrated in the above example but it showing error,
DependencyMap::Method “ApprovePrizeTest.fn_Approve()[pri:0, instance:testexecution.ApprovePrizeTest@4678c730]” depends on nonexistent group “createPrize”
at org.testng.DependencyMap.getMethodsThatBelongTo(DependencyMap.java:45)
at org.testng.TestRunner.createDynamicGraph(TestRunner.java:1079)
at org.testng.TestRunner.privateRun(TestRunner.java:734)
at org.testng.TestRunner.run(TestRunner.java:617)
at org.testng.SuiteRunner.runTest(SuiteRunner.java:334)
at org.testng.SuiteRunner.runSequentially(SuiteRunner.java:329)
at org.testng.SuiteRunner.privateRun(SuiteRunner.java:291)
at org.testng.SuiteRunner.run(SuiteRunner.java:240)
at org.testng.SuiteRunnerWorker.runSuite(SuiteRunnerWorker.java:52)
at org.testng.SuiteRunnerWorker.run(SuiteRunnerWorker.java:86)
at org.testng.TestNG.runSuitesSequentially(TestNG.java:1198)
at org.testng.TestNG.runSuitesLocally(TestNG.java:1123)
at org.testng.TestNG.run(TestNG.java:1031)
at org.testng.remote.AbstractRemoteTestNG.run(AbstractRemoteTestNG.java:114)
at org.testng.remote.RemoteTestNG.initAndRun(RemoteTestNG.java:251)
at org.testng.remote.RemoteTestNG.main(RemoteTestNG.java:77)
Hi Mkyong, I want RUN a test Method at the end (after all the test methods of the class are run). I tried putting “*” for dependsonmethod but it didnt like it, any work around?
Hey Mkyong, How can I control the dependency of a test based on Logical OR i.e. TestA should run if either if TestB OR TestC is passed? The one in your example is logical AND. Please suggest if there is any way for logical OR dependency methods. Thanks.
Mkyong,
Very clean explanation.!
One doubt: If I use two different testng files with one class each then in that case “dependsOnGroups” does not work..! Is it expected..? Is there any way I can depend on a method in different class + different testng file within same project..?
Hi mkyong, can you please tell me wich command did you use to execute the test suite in the testng.xml file
How to ignore dependency all methods at runtime…and example : test2 depends on test1 …I’m not able to skip test1 at runtime…awaiting for your response…could you tell me how to skip test 1 at runtime…
Thanks for sharing, i have one question. In a scenario, i have few tests with dependsOnMethods on a specific method and few other test-cases which are independent. What is the order of execution of test-cases in this scenario.
Thanks for providing good article on “TestNG – Dependency Test”. http://testing.blogercup.com/
Hi!! I have a different situation..I have two test methods and two data providers, the first test is working fine but the second test is giving “parameters unresolved” exception..
@DataProvider(name = “test1”)
public Object[][] DataProvider1()
{
……..
……
}
@Test(dataProvider = “test1”)
public void FnTest1String s1, String s2,String s3, String s4) throws InterruptedException
{
}
@DataProvider(name = “test2”)
public Object[][] DataProvider2()
{
……..
……
}
@Test(dataProvider = “test2”)
public void FnTest2(String s1, String s2,String s3, String s4) throws InterruptedException
{
}
Thanks for sharing this.
What happens if method1() has a parameter(s)? How would you specify this parameter in the dependsOnMethods annotation?
@Test
public void method1(int i) {
System.out.println(“This is method 1”);
}
How about if this method is in a different class?
Thanks again
1. Passing the parameter via @DataProvider, not dependsOnMethods
2. Create a testng.xml, suite test to test multiple test classes.
Thanks a ton , nw it s like plain vanila
you´re great man! TestNG rocks!
Thanks for your post.
I’m not sure when method1 be run.
Please help me know: When I call(or link) method1 by dependsOnMethods={“method1”}, it only check method1 be run/not run or system will run method1 at that time?
method1() is a test case as well. System will run method1(), if it is passed, method2() will be executed next. Refer to above scenario.
Hello,
Thanks for the post.
Now, suppose I want to execute/run method2 only.. How can I do that?? I tried adding annotation ignoreMissingDependencies=true but it throws exception
Thanks
Awaiting response!!
Somesh
If your method is able to run independent, why need to add the dependency?
Nice post
Hi,
I have just begun learning TestNG and this tutorial was indeed very helpful. Thank you.
I like the content
Hi,
Very good tutorials. I’m new to this and was wondering what to use, I think I’ll go with TestNG. Let me know if I understood correctly, TestNG includes all of JUnit4 + more features?
I’ve installed selenium and will install the TestNG plug-in, had some issues it didn’t find it, I’ll try again and see if it fixed it.
Thanks,
Azhar
Yes, TestNG includes all of JUnit4 + more features. The only problem is lack of TestNG resources available online.
very clear examples,very easy to understand.