Maven – How to create a Java web application project
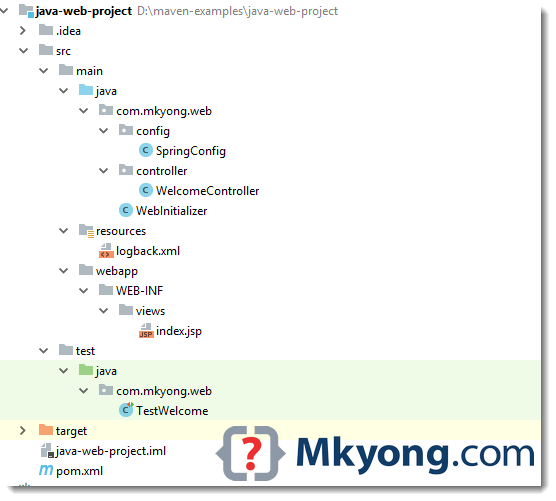
In this tutorial, we will show you how to use Maven to manage a Java web project. At the end, we will create a Spring MVC web application, display a current date on a JSP page.
Technologies used :
- Maven 3.5.3
- JDK 8
- Spring 5.1.0.RELEASE
- JUnit 5
- Logback 1.2.3
- Jetty 9.4.x or Tomcat 8.5
1. Create a web project from Maven Template
Create a web project from Maven template maven-archetype-webapp
mvn archetype:generate
-DgroupId={project-packaging}
-DartifactId={project-name}
-DarchetypeArtifactId={maven-template}
-DinteractiveMode=false
For example,
D:\>mvn archetype:generate -DgroupId=com.mkyong.web -DartifactId=java-web-project -DarchetypeArtifactId=maven-archetype-webapp -DinteractiveMode=false
[INFO] Scanning for projects...
[INFO]
[INFO] ------------------< org.apache.maven:standalone-pom >-------------------
[INFO] Building Maven Stub Project (No POM) 1
[INFO] --------------------------------[ pom ]---------------------------------
[INFO]
[INFO] >>> maven-archetype-plugin:3.0.1:generate (default-cli) > generate-sources @ standalone-pom >>>
[INFO]
[INFO] <<< maven-archetype-plugin:3.0.1:generate (default-cli) < generate-sources @ standalone-pom <<<
[INFO]
[INFO]
[INFO] --- maven-archetype-plugin:3.0.1:generate (default-cli) @ standalone-pom ---
[INFO] Generating project in Batch mode
[INFO] ----------------------------------------------------------------------------
[INFO] Using following parameters for creating project from Old (1.x) Archetype: maven-archetype-webapp:1.0
[INFO] ----------------------------------------------------------------------------
[INFO] Parameter: basedir, Value: D:\
[INFO] Parameter: package, Value: com.mkyong.web
[INFO] Parameter: groupId, Value: com.mkyong.web
[INFO] Parameter: artifactId, Value: java-web-project
[INFO] Parameter: packageName, Value: com.mkyong.web
[INFO] Parameter: version, Value: 1.0-SNAPSHOT
[INFO] project created from Old (1.x) Archetype in dir: D:\java-web-project
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 6.509 s
[INFO] Finished at: 2018-10-04T15:25:16+08:00
[INFO] ------------------------------------------------------------------------
Actually, this is optional to generate a web project from a Maven web template. You can always generate those folders with the classic
mkdir
command manually.
2. Maven Template
2.1 The following project directory structure will be created.
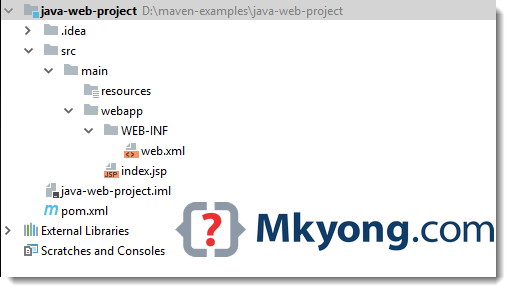
P.S Above figure is captured from IntelliJ IDEA, just ignore those IDE folders like .idea
and java-web-project.iml
2.2 Review the generated pom.xml
.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mkyong.web</groupId>
<artifactId>java-web-project</artifactId>
<packaging>war</packaging>
<version>1.0-SNAPSHOT</version>
<name>java-web-project Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<finalName>java-web-project</finalName>
</build>
</project>
P.S The generated files are not much value, we will update all of them later. First, delete the web.xml
, we don’t need this.
3. Update POM
3.1 Update the pom.xml
file, add dependencies for Spring MVC for web framework, JUnit for unit test, Jetty server to test the web project, and also some Maven configuration.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mkyong.web</groupId>
<artifactId>java-web-project</artifactId>
<packaging>war</packaging>
<version>1.0-SNAPSHOT</version>
<name>java-web-project Maven Webapp</name>
<url>http://maven.apache.org</url>
<properties>
<!-- https://maven.apache.org/general.html#encoding-warning -->
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<spring.version>5.1.0.RELEASE</spring.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- logging , spring 5 no more bridge, thanks spring-jcl -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
<!-- junit 5, unit test -->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.3.1</version>
<scope>test</scope>
</dependency>
<!-- unit test -->
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest-library</artifactId>
<version>1.3</version>
<scope>test</scope>
</dependency>
<!-- for web servlet -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!-- Some containers like Tomcat don't have jstl library -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>java-web-project</finalName>
<plugins>
<!-- http://www.eclipse.org/jetty/documentation/current/jetty-maven-plugin.html -->
<plugin>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-maven-plugin</artifactId>
<version>9.4.12.v20180830</version>
</plugin>
<!-- Default is too old, update to latest to run the latest Spring 5 + jUnit 5 -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.0</version>
</plugin>
<!-- Default 2.2 is too old, update to latest -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>3.2.2</version>
</plugin>
</plugins>
</build>
</project>
3.2 Display the project dependencies.
D:\> mvn dependency:tree
...
[INFO] --- maven-dependency-plugin:2.8:tree (default-cli) @ java-web-project ---
[INFO] com.mkyong.web:java-web-project:war:1.0-SNAPSHOT
[INFO] +- org.springframework:spring-webmvc:jar:5.1.0.RELEASE:compile
[INFO] | +- org.springframework:spring-aop:jar:5.1.0.RELEASE:compile
[INFO] | +- org.springframework:spring-beans:jar:5.1.0.RELEASE:compile
[INFO] | +- org.springframework:spring-context:jar:5.1.0.RELEASE:compile
[INFO] | +- org.springframework:spring-core:jar:5.1.0.RELEASE:compile
[INFO] | | \- org.springframework:spring-jcl:jar:5.1.0.RELEASE:compile
[INFO] | +- org.springframework:spring-expression:jar:5.1.0.RELEASE:compile
[INFO] | \- org.springframework:spring-web:jar:5.1.0.RELEASE:compile
[INFO] +- org.springframework:spring-test:jar:5.1.0.RELEASE:compile
[INFO] +- ch.qos.logback:logback-classic:jar:1.2.3:compile
[INFO] | +- ch.qos.logback:logback-core:jar:1.2.3:compile
[INFO] | \- org.slf4j:slf4j-api:jar:1.7.25:compile
[INFO] +- org.junit.jupiter:junit-jupiter-engine:jar:5.3.1:test
[INFO] | +- org.apiguardian:apiguardian-api:jar:1.0.0:test
[INFO] | +- org.junit.platform:junit-platform-engine:jar:1.3.1:test
[INFO] | | +- org.junit.platform:junit-platform-commons:jar:1.3.1:test
[INFO] | | \- org.opentest4j:opentest4j:jar:1.1.1:test
[INFO] | \- org.junit.jupiter:junit-jupiter-api:jar:5.3.1:test
[INFO] +- org.hamcrest:hamcrest-library:jar:1.3:test
[INFO] | \- org.hamcrest:hamcrest-core:jar:1.3:test
[INFO] +- javax.servlet:javax.servlet-api:jar:3.1.0:provided
[INFO] \- javax.servlet:jstl:jar:1.2:provided
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 0.931 s
[INFO] Finished at: 2018-10-08T15:55:08+08:00
[INFO] ------------------------------------------------------------------------
4. Spring MVC + JSP + LogBack
4.1 Create a few files to bootstrap Spring MVC web project.
package com.mkyong.web.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import org.springframework.web.servlet.view.InternalResourceViewResolver;
import org.springframework.web.servlet.view.JstlView;
@EnableWebMvc
@Configuration
@ComponentScan({"com.mkyong.web"})
public class SpringConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/resources/**")
.addResourceLocations("/resources/");
}
@Bean
public InternalResourceViewResolver viewResolver() {
InternalResourceViewResolver viewResolver
= new InternalResourceViewResolver();
viewResolver.setViewClass(JstlView.class);
viewResolver.setPrefix("/WEB-INF/views/");
viewResolver.setSuffix(".jsp");
return viewResolver;
}
}
package com.mkyong.web;
import com.mkyong.web.config.SpringConfig;
import org.springframework.web.servlet.support.AbstractAnnotationConfigDispatcherServletInitializer;
public class WebInitializer extends AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class<?>[] getRootConfigClasses() {
return null;
}
@Override
protected Class<?>[] getServletConfigClasses() {
return new Class[]{SpringConfig.class};
}
@Override
protected String[] getServletMappings() {
return new String[]{"/"};
}
}
package com.mkyong.web.controller;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import java.util.Date;
@Controller
public class WelcomeController {
private final Logger logger = LoggerFactory.getLogger(WelcomeController.class);
@GetMapping("/")
public String index(Model model) {
logger.debug("Welcome to mkyong.com...");
model.addAttribute("msg", getMessage());
model.addAttribute("today", new Date());
return "index";
}
private String getMessage() {
return "Hello World";
}
}
4.2 Move the index.jsp
file into the WEB-INF
folder, and update it
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt"%>
<html>
<body>
<h1>${msg}</h1>
<h2>Today is <fmt:formatDate value="${today}" pattern="yyy-MM-dd" /></h2>
</body>
</html>
4.3 Logs to console.
<?xml version="1.0" encoding="UTF-8"?>
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<layout class="ch.qos.logback.classic.PatternLayout">
<Pattern>
%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n
</Pattern>
</layout>
</appender>
<logger name="com.mkyong.web" level="debug"
additivity="false">
<appender-ref ref="STDOUT"/>
</logger>
<root level="error">
<appender-ref ref="STDOUT"/>
</root>
</configuration>
5. Unit Test
A simple Spring MVC 5 + JUnit 5 example.
package com.mkyong.web;
import com.mkyong.web.config.SpringConfig;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.junit.jupiter.web.SpringJUnitWebConfig;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import org.springframework.web.context.WebApplicationContext;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultHandlers.print;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
@SpringJUnitWebConfig(SpringConfig.class)
public class TestWelcome {
private MockMvc mockMvc;
@Autowired
private WebApplicationContext webAppContext;
@BeforeEach
public void setup() {
mockMvc = MockMvcBuilders.webAppContextSetup(webAppContext).build();
}
@Test
public void testWelcome() throws Exception {
this.mockMvc.perform(
get("/"))
.andDo(print())
.andExpect(status().isOk())
.andExpect(view().name("index"))
.andExpect(forwardedUrl("/WEB-INF/views/index.jsp"))
.andExpect(model().attribute("msg", "Hello World"));
}
}
6. Directory Structure
Review the final files and directory structure.
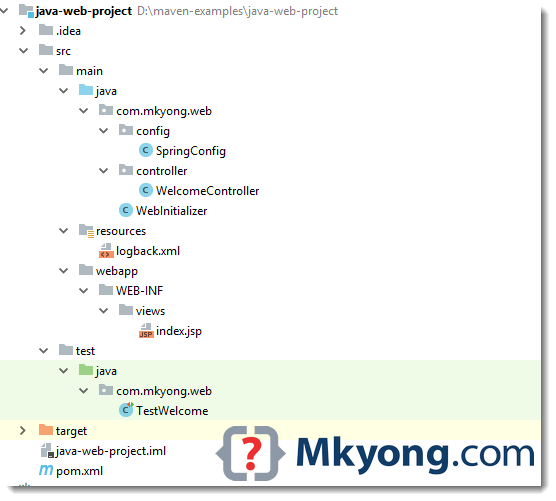
Read this Maven Standard Directory Layout.
7. Demo
7.1 Test the web project with Jetty web server – mvn jetty:run
D:\> mvn jetty:run
[INFO] webAppSourceDirectory not set. Trying src\main\webapp
[INFO] Reload Mechanic: automatic
[INFO] nonBlocking:false
[INFO] Classes = D:\java-web-project\target\classes
[INFO] Configuring Jetty for project: java-web-project Maven Webapp
[INFO] Logging initialized @4821ms to org.eclipse.jetty.util.log.Slf4jLog
[INFO] Context path = /
[INFO] Tmp directory = D:\java-web-project\target\tmp
[INFO] Web defaults = org/eclipse/jetty/webapp/webdefault.xml
[INFO] Web overrides = none
[INFO] web.xml file = null
[INFO] Webapp directory = D:\java-web-project\src\main\webapp
[INFO] jetty-9.4.12.v20180830; built: 2018-08-30T13:59:14.071Z; git: 27208684755d94a92186989f695db2d7b21ebc51; jvm 10.0.1+10
...
[INFO] 1 Spring WebApplicationInitializers detected on classpath
2018-10-08 15:11:50 [main] DEBUG com.mkyong.web.WebInitializer - No ContextLoaderListener registered, as createRootApplicationContext() did not return an application context
[INFO] DefaultSessionIdManager workerName=node0
[INFO] No SessionScavenger set, using defaults
[INFO] node0 Scavenging every 660000ms
[INFO] Initializing Spring DispatcherServlet 'dispatcher'
[INFO] Started o.e.j.m.p.JettyWebAppContext@68a78f3c{/,file:///D:/java-web-project/src/main/webapp/,AVAILABLE}{file:///D:/java-web-project/src/main/webapp/}
[INFO] Started ServerConnector@3355168{HTTP/1.1,[http/1.1]}{0.0.0.0:8080}
[INFO] Started @6271ms
[INFO] Started Jetty Server
2018-10-08 15:12:01 [qtp1373051324-19] DEBUG c.m.web.controller.WelcomeController - Welcome to mkyong.com...
7.2 Access it via http://localhost:8080/
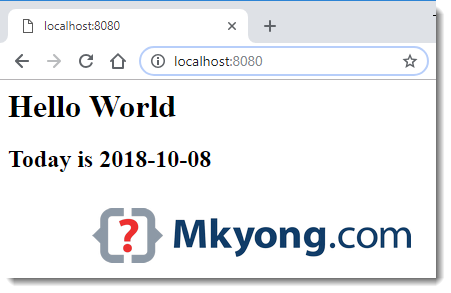
P.S CTRL + C to stop the Jetty web server.
8. Deployment
8.1 mvn package
to generate a WAR file for deployment.
D:\> mvn package
...
[INFO] Packaging webapp
[INFO] Assembling webapp [java-web-project] in [D:\java-web-project\target\java-web-project]
[INFO] Processing war project
[INFO] Copying webapp resources [D:\java-web-project\src\main\webapp]
[INFO] Webapp assembled in [89 msecs]
[INFO] Building war: D:\java-web-project\target\java-web-project.war
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 3.844 s
[INFO] Finished at: 2018-10-08T15:31:12+08:00
[INFO] ------------------------------------------------------------------------
The default directory for the generated WAR is target/finalName
. Done.
Download Source Code
$ cd java-web-project
$ mvn jetty:run
http://localhost:8080
Wonderful! my first runnable spring mvc
[INFO] ————————————————————-
[ERROR] COMPILATION ERROR :
[INFO] ————————————————————-
[ERROR] /Users/i844276/Downloads/LINUX-CODING-VIEW/JavaProgram/mvnProjectExample/maven-examples/java-web-project/src/main/java/com/mkyong/web/WebInitializer.java:[3,29] package com.mkyong.web.config does not exist
[ERROR] /Users/i844276/Downloads/LINUX-CODING-VIEW/JavaProgram/mvnProjectExample/maven-examples/java-web-project/src/main/java/com/mkyong/web/WebInitializer.java:[15,28] cannot find symbol
symbol: class SpringConfig
location: class com.mkyong.web.WebInitializer
[INFO] 2 errors
Hi,
I had a run compilation error due the “mvn tomcat:run” started Tomcat 6 but the classes are in Java 7.
A quick fix: use tomcat7:run
It could happen because I have installed tomcat6 and 7.
FYI this the error if you need to use “mvn tomcat7:run” instead of “mvn tomcat:run”:
org.apache.jasper.JasperException: Unable to compile class for JSP:
An error occurred at line: 1 in the generated java file
The type java.io.ObjectInputStream cannot be resolved. It is indirectly referenced from required .class files
Stacktrace:
org.apache.jasper.compiler.DefaultErrorHandler.javacError(DefaultErrorHandler.java:92)
Thank you Rafael!
I think this example is now dated and no longer works properly. I spent a lot of time trying to get it working but eventually gave up. 🙁
hi guys
Changes (step by step) for running the project on Tomcat:
[1] “mvn tomcat:run” – “Apache Tomcat/6.0.29” could not start because “address already in use” error happened for default port 8080
[2] “mvn tomcat:run -Dmaven.tomcat.port=9999” – “Apache Tomcat/6.0.29” started, but “war” running on “http://localhost:9999/java-web-project” caused the error “HTTP Status 404 – /java-web-project/” with description “The requested resource (/java-web-project/) is not available.”
[3] “mvn tomcat7:run -Dmaven.tomcat.port=9999” – “Apache Tomcat/7.0.47” started after adding plugin in POM’s plugins section:
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.2</version>
</plugin>
but “war” running on “http://localhost:9999/java-web-project” caused the error:
“HTTP Status 500 – Handler processing failed; nested exception is java.lang.NoClassDefFoundError: javax/servlet/jsp/jstl/core/Config” with description “The server encountered an internal error that prevented it from fulfilling this request.”
[4] “mvn tomcat7:run -Dmaven.tomcat.port=9999” – “Apache Tomcat/7.0.47” started after commenting out “provided” scope in “jstl” dependency:
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
<!– <scope>provided</scope> –>
</dependency>
according to the remark above it “<!– Some containers like Tomcat don’t have jstl library –>”
and finally “war” running on “http://localhost:9999/java-web-project” displayed:
Hello WorldToday is 2021-07-11
BTW#1, I have used the following environment settings: “Apache Maven 3.8.1″, “Java version: 1.8.0_202”, “OS name: windows 10” and “Eclipse 2020-12”.
BTW#2…
“config/SpringConfig.java” file is missing in GitHub repository:
“https://github.com/mkyong/maven-examples/tree/master/java-web-project/src/main/java/com/mkyong/web”
the above maven web application is not compatible with Tomcat 10 but it is perfectly running on tomcat 9.
any solution for that?
I am having the most hardest time getting this war onto a Dockerfile based on Jetty. The result being INFO:oejshC.root:main: No Spring WebApplicationInitializer types detected on classpath
There’s a lot of helpful material on this forum. Thanks Mykong
If you’re getting an error of
“unable to convert string [${today}] to class [java.util.Date] for attribute [value]: [Property Editor not registered with the PropertyEditorManager]”
then the solution is to edit your web.xml as follows:
1) Remove the doctype line at the top.
2) Add the following
(Note this is inside the web-app tag)
Hello mkyong, I am getting the below error when trying to generate the project structure.
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-archetype-plugin:3.0.1:generate (default-cli) on project standalone-pom: Execution default-cli of goal org.apache.maven.plugins:maven-archetype-plugin:3.0.1:generate failed: A required class was missing while executing org.apache.maven.plugins:maven-archetype-plugin:3.0.1:generate: org/apache/commons/lang/StringUtils
Failed to execute goal org.apache.maven.plugins:maven-archetype-plugin:3.0.1:generate class not foundStringUtils
really awesome, please do add some extra things for the next time
Worked perfectly in 2017. Thank you sir!
Firstly I would like to thank you for sharing the knowledge. I have a doubt here after updating the pom.xml with the required dependencies and plugin i ran the command “mvn eclipse:eclipse” ideally it should create all the folders and the required xmls(logback,mvc dispacther etc) in the folders path. But it is not happening for me. Kindly provide your help here.
Hi,
I am seeing this error once i imported this project in to my eclipse in mvc-dispatcherservlet(even after doing updatemavenproject.
The errors below were detected when validating the file “spring-beans.xsd” via the file “mvc-dispatcher-servlet.xml”. In most cases these errors can be detected by validating “spring-beans.xsd” directly. However it is possible that errors will only occur when spring-beans.xsd is validated in the context of mvc-dispatcher-servlet.xml.
Thank You so much…this is the best beginner tutorial I have come across till now to create a simple web application. Thanks again to help me get started.
Please, don’t confuse “Web application projects” with “Web apps” that can be created with services as http://www.socialcreator.com 😉
Imported project into Spring Tools Suite and index.jsp has the following error notification.
Multiple annotations found at this line:
– The superclass “javax.servlet.http.HttpServlet” was not found on the Java Build
Path
– The superclass “javax.servlet.http.HttpServlet” was not found on the Java Build
Path
I see that the javax jars are under referenced libraries – jstl-1.2.jar.
why does index not see these dependencies?
I too have the same error. How to solve this? Please help me I am new to Maven
i have a doubt..hot deployment will happen when we run using maven right?
Just downloaded your code and got an error about missing ddl in the config file for logback.
See this for suggestions: http://stackoverflow.com/questions/5731162/xml-schema-or-dtd-for-logback-xml
BTW: Thanks for the concise and illustrative example.
how to develop a maven web project without using spring sir.
ITs really helpful blog.
Hi Mkyong , thanks for the tutorial but it has that missing dependency in pom.xml
org.apache.tomcat
tomcat-servlet-api
7.0.30
provided
or it can run on eclipse by adding tomcat 7 runtime.
Thanks a lot. Mkyong rocks!
counter is not working help
Thanks for your advice.
Pls do not type command directly to your command prompt if you want a maven webapp
you will need to specified the archetype as webapp i had few issue with the example given on top that said type mvn archetype:generate -DgroupId={project-packaging} -DartifactId={project-name} -DarchetypeArtifactId=maven-archetype-webapp -DinteractiveMode=false
*** Again change the archetype to webapp if you want a web application or will wind up with a jar files
I got it! Thank you for short explanation 😀
We have same problem here, but I still don’t understand your solution. Where should I change the archetype again?
Decided to finally say ‘thank you’ as well.
This is sooo what I searched for. The most useful starter guide ever.
Thank you very much for this part of work, as well as for all other great articles.
Hi,
I’ve followed this tutorial and looked at other related tutorials you’ve posted all of which have been very helpful in getting me started. However I have an error in tomcat. The startup of the server and deploy using maven runs without errors and I have no errors on my tomcat logs but when trying to access my project I receive a 404 status from tomcat. Do you have any suggestions please?
Regards,
Lisa Young
Thanks so much for these awesome guides. Your tutorials over spring, jdbc, maven, and now tomcat have helped me a tremendous amount.