PrimeFaces – Open Window By Dropdown Selection
Technology: Primefaces it’s java based web framework to develop web applications using java.
It’s one of the compliance frameworks for JSF, there are many other frameworks like omnifaces, richfaces. Primefaces has rich UI components.
In this tutorial primefaces, maven, java8, glasifish servers are used.
Use case: If you want to open a new page by clicking on button, link or something it’s straight forward thing. Even if the page URL is addressed from directly backing bean we can do it. But if the page URL is dynamic, it’s not a straight forward thing because by the time you click on the button, the page is already rendered, so you will have old value. So, in this case I am going to implement this use case.
There are several steps to implement this,
- Create a Primefaces maven project, download glassfish and add it in the server part of eclipse.
- Deploy the application and test.
1. Project Structure
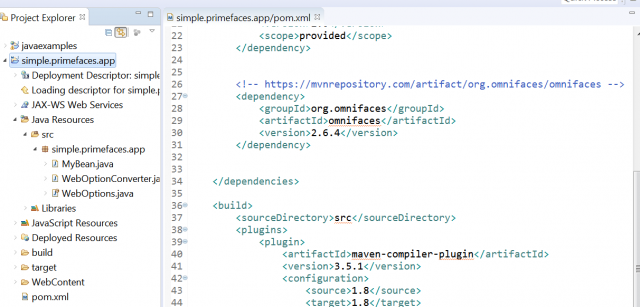
2. Project Dependencies
I just added primefaces, jsf library.
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>simple.primefaces.app</groupId>
<artifactId>simple.primefaces.app</artifactId>
<version>2017.09.01</version>
<packaging>war</packaging>
<name>primefaces-app</name>
<dependencies>
<!-- https://mvnrepository.com/artifact/org.primefaces/primefaces -->
<dependency>
<groupId>org.primefaces</groupId>
<artifactId>primefaces</artifactId>
<version>5.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.faces/jsf-api -->
<dependency>
<groupId>javax.faces</groupId>
<artifactId>jsf-api</artifactId>
<version>2.0</version>
<scope>provided</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.omnifaces/omnifaces -->
<dependency>
<groupId>org.omnifaces</groupId>
<artifactId>omnifaces</artifactId>
<version>2.6.4</version>
</dependency>
</dependencies>
<build>
<sourceDirectory>src</sourceDirectory>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.5.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<plugin>
<artifactId>maven-war-plugin</artifactId>
<version>3.0.0</version>
<configuration>
<warSourceDirectory>WebContent</warSourceDirectory>
</configuration>
</plugin>
</plugins>
</build>
</project>
3. Code
Have created a Bean, Converter and Enum and index.xhtml(facelet)
package simple.primefaces.app;
import java.util.Arrays;
import java.util.List;
import javax.faces.bean.ManagedBean;
@ManagedBean
public class MyBean {
private static final String GOOGLE = "https://www.google.co.in/";
private static final String YAHOO = "https://in.yahoo.com/";
private WebOptions selectedOption;
private String webOptionUrl;
public String getWebOptionUrl() {
return webOptionUrl;
}
public void setWebOptionUrl(String webOptionUrl) {
this.webOptionUrl = webOptionUrl;
}
public void prepareUrl(){
if(WebOptions.GOOGLE.equals(selectedOption)){
webOptionUrl = GOOGLE;
} else if(WebOptions.YAHOO.equals(selectedOption)) {
webOptionUrl = YAHOO;
} else {
webOptionUrl = "";
}
}
public List<WebOptions> getAllWebOptions() {
return Arrays.asList(WebOptions.values());
}
public WebOptions getSelectedOption() {
return selectedOption;
}
public void setSelectedOption(WebOptions selectedOption) {
this.selectedOption = selectedOption;
}
}
package simple.primefaces.app;
import java.io.Serializable;
import javax.faces.component.UIComponent;
import javax.faces.context.FacesContext;
import javax.faces.convert.Converter;
import javax.faces.convert.FacesConverter;
import org.omnifaces.cdi.ViewScoped;
@FacesConverter(value = "simple.primefaces.app.WebOptionConverter", forClass = WebOptionConverter.class)
@ViewScoped
public class WebOptionConverter implements Converter, Serializable {
/** the serialVersionUID **/
private static final long serialVersionUID = -218581226063576481L;
public WebOptionConverter(){
super();
}
@Override
public Object getAsObject(FacesContext context, UIComponent component, String value) {
return WebOptions.getOptionByOptoin(value);
}
@Override
public String getAsString(FacesContext context, UIComponent component, Object value) {
if(value instanceof WebOptions){
final WebOptions objectStatus = (WebOptions) value;
return objectStatus.getWebOption();
}
return "";
}
}
package simple.primefaces.app;
public enum WebOptions {
GOOGLE("google"),
YAHOO("yahoo");
private String webOption;
WebOptions(String webOption){
this.webOption = webOption;
}
public static WebOptions getOptionByOptoin(String value){
if(WebOptions.GOOGLE.webOption.equals(value)){
return WebOptions.GOOGLE;
}
return WebOptions.YAHOO;
}
public String getWebOption() {
return webOption;
}
}
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
xmlns:ui="http://java.sun.com/jsf/facelets"
xmlns:p="http://primefaces.org/ui">
<h:head>
<script type="text/javascript">
function openUrl(){
var webOptionUrl = document.getElementById("websiteForm:webOptionHidden").value;
if(webOptionUrl.length === 0){
var message = 'Please select website';
alert(message);
} else {
window.open(webOptionUrl, '', 'width=1024, height=720, status=no, scrollbars=1 menubar=no, toolbar=no');
}
}
</script>
</h:head>
<h:body>
<h:form id="websiteForm">
<p:panelGrid id="selectOption" style="margin-left:500px;">
<p:row>
<p:column>
<p:outputLabel value="Please select website: "></p:outputLabel>
<p:selectOneMenu id="option" value="#{myBean.selectedOption}">
<f:selectItem itemLabel="--" itemValue="" />
<f:selectItems value="#{myBean.allWebOptions}" var="o" itemLabel="#{o.webOption}" itemValue="#{o}"/>
<p:ajax update="@form" process="@this" listener="#{myBean.prepareUrl}"></p:ajax>
<f:converter converterId="simple.primefaces.app.WebOptionConverter" />
</p:selectOneMenu>
</p:column>
</p:row>
<p:row>
<p:column>
<p:commandButton id="submitButton" value="Submit" oncomplete="openUrl();" update="webOptionHidden"/>
<p:inputText id="webOptionHidden" value="#{myBean.webOptionUrl}" style="display:none;"/>
</p:column>
</p:row>
</p:panelGrid>
</h:form>
</h:body>
</html>
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd"
id="WebApp_ID" version="3.0">
<display-name>simple.primefaces.app</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.xhtm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<context-param>
<param-name>com.sun.faces.enableRestoreView11Compatibility</param-name>
<param-value>true</param-value>
</context-param>
</web-app>
4, Demo
Build the application and add it to the server and run the application.
4.1 If you run the application, the screen will look like this,
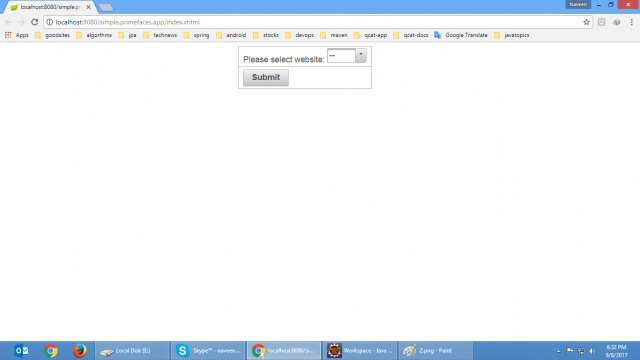
4.2 If you don’t select any option, it will prompt you to select an option.
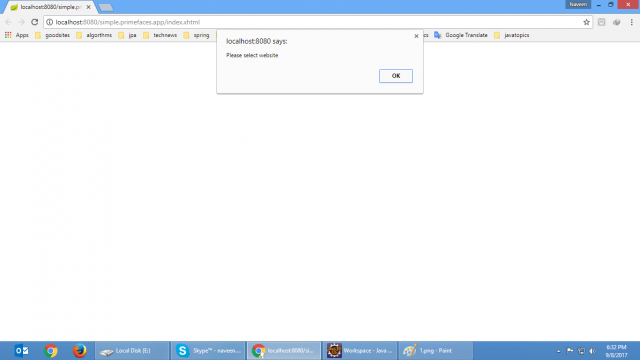
4.3 For now I have added two pages google and yahoo, if you select any of them, it’s corresponding website will open in another window.
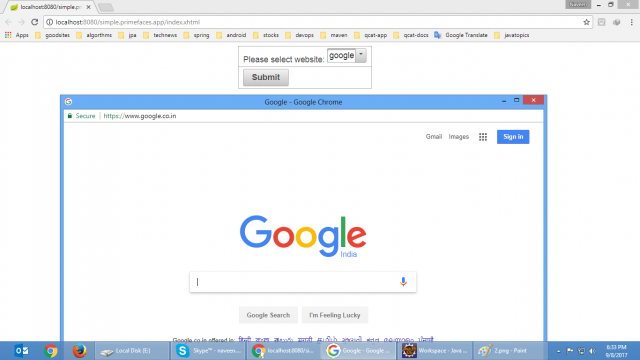
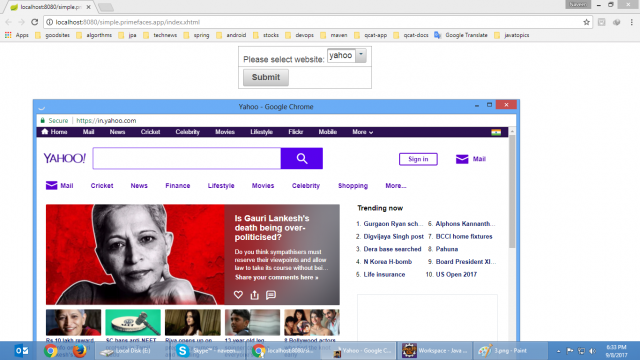
4.4 Now I will explain the trick, hope you are familiar with primefaces widgets, omnifaces conveter, backing bean… If you are not, I recommend you please go through primefaces tutorial.
- Let’s say didn’t select any option and clicked on submit, javascript method will be checked where I am checking whether any option is selected or not, if nothing selected just simply asking the user select any of the options.
- Let’s say you selected any of the options, now an Ajax call we invoked, causes the selected option will set to the backing bean and prepares it’s respective page URL. After that, (if you observe facelet, index.xhtml I have added a text field which will not be shown on the web page)
- After ajax call completed, this text field will be updated, I mean this text field will have the selected web option.
- If you click on the submit now, again the javascript method will be called, there we are taking the value from this text field, so it contains the URL of the option. So the URL will be opened in new window.


Experts Java development programmers team have shared their best knowledge about java technology and its use in java project. If you need to get more information, you can ask the developers who are already applying this technology in their projects.
Read More Related This :
JPA optimistic lock exception in Java Development
This post explains the JPA technology and its use in java development. Experts of java development India are explaining the use case of technologies- JPA and Hibernate, MySql database, Maven. Read this post and know what they want to say.
The page is web.xml and not Web.xhtml right ?
Nice Article…thanks for sharing