JSF 2 param example
In JSF, “f:param” tag allow you to pass a parameter to a component, but it’s behavior is different depends on which type of component it’s attached. For example,
1. f:param + h:outputFormat
If attach a “f:param” tag to “h:outputFormat“, the parameter is specifies the placeholder.
<h:outputFormat value="Hello,{0}. You are from {1}.">
<f:param value="JSF User" />
<f:param value="China" />
</h:outputFormat>
Here’s the output – “Hello JSF User. You are from China“.
2. f:param + Other Component
If you attach a “f:param” tag to other components like “h:commandButton” , the parameter is turned into request parameter.
<h:commandButton id="submitButton"
value="Submit - US" action="#{user.outcome}">
<f:param name="country" value="China" />
</h:commandButton>
In user bean, you can get back the parameter value like this :
Map<String,String> params =
FacesContext.getExternalContext().getRequestParameterMap();
String countrry = params.get("country");
JSF f:param example
Here’s a JSF 2.0 application, to show the use of f:param tag in both “h:commandButton” and “h:outputFormat” componenets.
1. Managed Bean
A simple managed bean.
UserBean.java
package com.mkyong;
import java.util.Map;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
import javax.faces.context.FacesContext;
@ManagedBean(name="user")
@SessionScoped
public class UserBean{
public String name;
public String country;
public String outcome(){
FacesContext fc = FacesContext.getCurrentInstance();
this.country = getCountryParam(fc);
return "result";
}
//get value from "f:param"
public String getCountryParam(FacesContext fc){
Map<String,String> params = fc.getExternalContext().getRequestParameterMap();
return params.get("country");
}
//getter and setter methods
}
2. JSF Page
Two JSF pages for demonstration.
default.xhtml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
>
<h:body>
<h1>JSF 2 param example</h1>
<h:form id="form">
Enter your name :
<h:inputText size="10" value="#{user.name}" />
<br /><br />
<h:commandButton id="submitButton"
value="Submit - US" action="#{user.outcome}">
<f:param name="country" value="United States" />
</h:commandButton>
</h:form>
</h:body>
</html>
result.xhtml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
>
<h:body>
<h1>JSF 2 param example</h1>
<h2>
<h:outputFormat value="Hello,{0}. You are from {1}.">
<f:param value="#{user.name}" />
<f:param value="#{user.country}" />
</h:outputFormat>
</h2>
</h:body>
</html>
3. Demo
Enter your name, e.g “mkyong”, and click on the button.
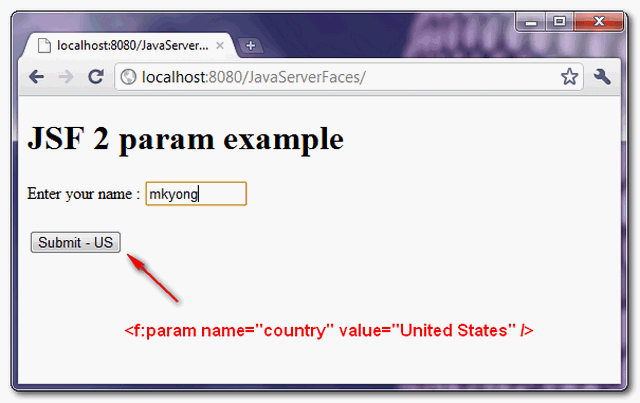
Display the formatted message, “name” from user input, “country” from button parameter.
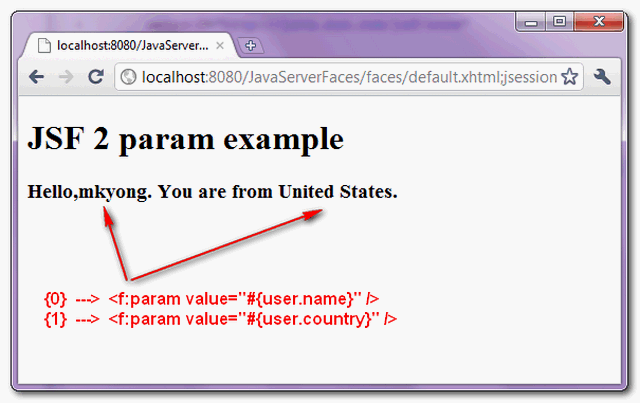
el parametro puede ser int o float?
If we put country property in another managed bean it refers to null !
@ManagedBean
@RequestScoped
public class SecBean {
@ManagedProperty(value=”#{param.country}”)
public String country;
public String getCountry() {
FacesContext fc = FacesContext.getCurrentInstance();
if(getCountryParam(fc)!=null)
return getCountryParam(fc);
else return country;
}
public void setCountry(String country) {
this.country = country;
}
//get value from “f:param”
public String getCountryParam(FacesContext fc){
Map params = fc.getExternalContext().getRequestParameterMap();
return params.get(“country”);
}
}
any suggestions ?
Great!
Thanks! Your post saved my day!
hi,
it is possible to get tag data(or html full page contain with text,image) from FacesContext in jsf
tag data mean body tag,head tag etc. actually i need that data to print page from backingbean page. please give some good suggestion for that. i posted my question also here
Link :- http://stackoverflow.com/questions/15810865/print-current-html-page-on-printer-from-java-bean-in-jsf
can i do this????
hi, the example is working but when i do that:
JSF 2 param example
its not working {user.country}” why????? help me please
thanks alot.
Hi.
I’m doing something similar and I think your code is very close to my needs.
I want to get values like you do (but without using f:param) and into a ui:repeat.
Like this:
Do you know the way to obtain this values???
Thanks
Hi,
thanks for the post,
I am trying to run the example doing mvn tomcat:run, and this exception happens:
Servlet /JavaServerFaces threw load() exception
java.lang.ClassCastException: javax.faces.webapp.FacesServlet cannot be cast to javax.servlet.Servlet
I don’t know what I can do, can you help me??
thanks again,
Juan
@Juan: Hi, did you find a solution to your problem ? I am getting the same error..
thanx
Laci
Comment out the servlet-api dependency in the pom.xml, it’s already provided by Tomcat.
yes it working..thanks a lot
omg! You saved my life. Thanks a million
Hi Cyril
Thank you. That exactly solved my issue. =)
Best regards,
Semo
Hi, this page helps me a lot. Tks.