Hibernate – One-to-One example (Annotation)
In this tutorial, it will reuse the entire infrastructure of the previous “Hibernate one to one relationship example – XML mapping” tutorial, enhance it to support Hibernate / JPA annotation.
Project Structure
See the final project structure of this tutorial.
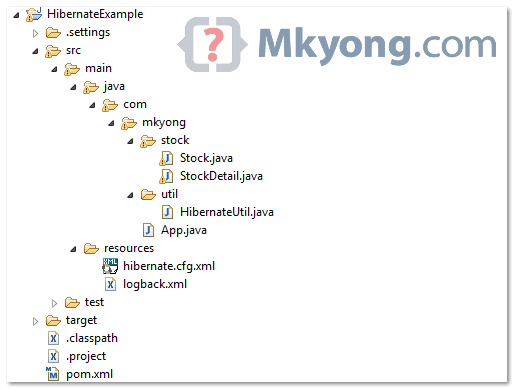
Note
Since Hibernate 3.6, annotation codes are merged into the Hibernate core module, so, the “previous pom.xml file can be reuse.
Since Hibernate 3.6, annotation codes are merged into the Hibernate core module, so, the “previous pom.xml file can be reuse.
1. “One-to-one” table relationship
See the previous one to one table relationship again.
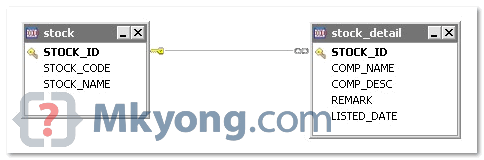
2. Hibernate Model Class
Create two model classes – Stock.java
and StockDetail.java
, and put the annotation code inside.
File : Stock.java
package com.mkyong.stock;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import static javax.persistence.GenerationType.IDENTITY;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.Table;
import javax.persistence.UniqueConstraint;
@Entity
@Table(name = "stock", catalog = "mkyongdb", uniqueConstraints = {
@UniqueConstraint(columnNames = "STOCK_NAME"),
@UniqueConstraint(columnNames = "STOCK_CODE") })
public class Stock implements java.io.Serializable {
private Integer stockId;
private String stockCode;
private String stockName;
private StockDetail stockDetail;
public Stock() {
}
public Stock(String stockCode, String stockName) {
this.stockCode = stockCode;
this.stockName = stockName;
}
public Stock(String stockCode, String stockName, StockDetail stockDetail) {
this.stockCode = stockCode;
this.stockName = stockName;
this.stockDetail = stockDetail;
}
@Id
@GeneratedValue(strategy = IDENTITY)
@Column(name = "STOCK_ID", unique = true, nullable = false)
public Integer getStockId() {
return this.stockId;
}
public void setStockId(Integer stockId) {
this.stockId = stockId;
}
@Column(name = "STOCK_CODE", unique = true, nullable = false, length = 10)
public String getStockCode() {
return this.stockCode;
}
public void setStockCode(String stockCode) {
this.stockCode = stockCode;
}
@Column(name = "STOCK_NAME", unique = true, nullable = false, length = 20)
public String getStockName() {
return this.stockName;
}
public void setStockName(String stockName) {
this.stockName = stockName;
}
@OneToOne(fetch = FetchType.LAZY, mappedBy = "stock", cascade = CascadeType.ALL)
public StockDetail getStockDetail() {
return this.stockDetail;
}
public void setStockDetail(StockDetail stockDetail) {
this.stockDetail = stockDetail;
}
}
File : StockDetail.java
package com.mkyong.stock;
import java.util.Date;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.PrimaryKeyJoinColumn;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
import org.hibernate.annotations.GenericGenerator;
import org.hibernate.annotations.Parameter;
@Entity
@Table(name = "stock_detail", catalog = "mkyongdb")
public class StockDetail implements java.io.Serializable {
private Integer stockId;
private Stock stock;
private String compName;
private String compDesc;
private String remark;
private Date listedDate;
public StockDetail() {
}
public StockDetail(Stock stock, String compName, String compDesc,
String remark, Date listedDate) {
this.stock = stock;
this.compName = compName;
this.compDesc = compDesc;
this.remark = remark;
this.listedDate = listedDate;
}
@GenericGenerator(name = "generator", strategy = "foreign",
parameters = @Parameter(name = "property", value = "stock"))
@Id
@GeneratedValue(generator = "generator")
@Column(name = "STOCK_ID", unique = true, nullable = false)
public Integer getStockId() {
return this.stockId;
}
public void setStockId(Integer stockId) {
this.stockId = stockId;
}
@OneToOne(fetch = FetchType.LAZY)
@PrimaryKeyJoinColumn
public Stock getStock() {
return this.stock;
}
public void setStock(Stock stock) {
this.stock = stock;
}
@Column(name = "COMP_NAME", nullable = false, length = 100)
public String getCompName() {
return this.compName;
}
public void setCompName(String compName) {
this.compName = compName;
}
@Column(name = "COMP_DESC", nullable = false)
public String getCompDesc() {
return this.compDesc;
}
public void setCompDesc(String compDesc) {
this.compDesc = compDesc;
}
@Column(name = "REMARK", nullable = false)
public String getRemark() {
return this.remark;
}
public void setRemark(String remark) {
this.remark = remark;
}
@Temporal(TemporalType.DATE)
@Column(name = "LISTED_DATE", nullable = false, length = 10)
public Date getListedDate() {
return this.listedDate;
}
public void setListedDate(Date listedDate) {
this.listedDate = listedDate;
}
}
3. Hibernate Configuration File
Puts annotated classes Stock.java
and StockDetail.java
in your Hibernate configuration file, and also MySQL connection details.
File : hibernate.cfg.xml
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mkyongdb</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">password</property>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="show_sql">true</property>
<mapping class="com.mkyong.stock.Stock" />
<mapping class="com.mkyong.stock.StockDetail" />
</session-factory>
</hibernate-configuration>
4. Run It
Run it, Hibernate will insert a row into the STOCK table and a row into the STOCK_DETAIL table.
File : App.java
package com.mkyong;
import java.util.Date;
import org.hibernate.Session;
import com.mkyong.stock.Stock;
import com.mkyong.stock.StockDetail;
import com.mkyong.util.HibernateUtil;
public class App {
public static void main(String[] args) {
System.out.println("Hibernate one to one (Annotation)");
Session session = HibernateUtil.getSessionFactory().openSession();
session.beginTransaction();
Stock stock = new Stock();
stock.setStockCode("7052");
stock.setStockName("PADINI");
StockDetail stockDetail = new StockDetail();
stockDetail.setCompName("PADINI Holding Malaysia");
stockDetail.setCompDesc("one stop shopping");
stockDetail.setRemark("vinci vinci");
stockDetail.setListedDate(new Date());
stock.setStockDetail(stockDetail);
stockDetail.setStock(stock);
session.save(stock);
session.getTransaction().commit();
System.out.println("Done");
}
}
Output
Hibernate one to one (Annotation)
Hibernate: insert into mkyongdb.stock (STOCK_CODE, STOCK_NAME) values (?, ?)
Hibernate: insert into mkyongdb.stock_detail
(COMP_DESC, COMP_NAME, LISTED_DATE, REMARK, STOCK_ID) values (?, ?, ?, ?, ?)
Done
Download it – Hibernate-one-to-one-annotation,zip (9KB)
is it possible to entered both annotation onetomany and manytoone in a single entity with hibernate annotation because i have three table state, district and block (all of have onetomany relation state-district-block but always have an error district.block is not mapped by
any one please help me
Thanks for the article.
Well, but how to StockDetail LAZY LOADING, please
THIS CODE IS BAD, beacause why when : “FROM Stock a”; This query get ALL DATA i.e Stock -> StockDetail (get data), it is bad “@OneToOne(fetch = FetchType.LAZY,” NOT WORK NOT WORK NOT WORK
I am new to Hibernate , I started one application using spring with Hibernate annotations,
I got strucked with errors, please guide me,
Caused by: org.hibernate.MappingException: Could not determine type for:
Actually I have one table in that I have two foreign keys, from two different tables,
How can I map those two tables? please guide me, if u want anything more , please mail me,
my mail id :[email protected]
Hi,
Thank you for your post and your good job on your site. It’s a pleasure to read you.
I tried your example. It seems that lazy loading doesn’t work when reading step.
I found this :
http://stackoverflow.com/questions/4653909/hibernate-jpa-onetoone-querying-despite-lazy-fetch
But I didn’t make working yet…
Best regards,
Thierry
In my case, this says that
“javax.persistence.PersistenceException: org.hibernate.exception.GenericJDBCException: Field ‘stockId’ doesn’t have a default value”
Here ‘stockId’ is field of stockDetail.
Also data is being inserted only in ‘stock’ and while inserting in ‘stockDetail’ it shows this exception.
What should I do?
I’m getting
Exception in thread “main” org.hibernate.exception.SQLGrammarException: could not execute statement
at org.hibernate.exception.internal.SQLExceptionTypeDelegate.convert(SQLExceptionTypeDelegate.java:80)
at org.hibernate.exception.internal.StandardSQLExceptionConverter.convert(StandardSQLExceptionConverter.java:49)
at org.hibernate.engine.jdbc.spi.SqlExceptionHelper.convert(SqlExceptionHelper.java:126)
at org.hibernate.engine.jdbc.spi.SqlExceptionHelper.convert(SqlExceptionHelper.java:112)
at org.hibernate.engine.jdbc.internal.ResultSetReturnImpl.executeUpdate(ResultSetReturnImpl.java:211)
Caused by: com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: Table ‘test.stock’ doesn’t exist
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:57)
I have de same configuration on hbm, but.. the result is :
Hibernate:
insert into TLMS005_CONTRATO
(CD_CLIENTE, CD_CUENTA, NB_PRODUCTO, CD_FOLIODIGIT,
TM_RECEPCION, CD_ESTATUS, CD_CONTRATO) values (?, ?, ?, ?, ?,
?, ?)
Hibernate:
update TLMS004_RECUARCH set
TP_ARCHIVO=?,
NB_RUTAARCH=?,
TX_MSGERR=?,
TM_ACTUALIZACION=?,
ST_RECUPERACION=?
where
CD_CONTRATO=?
why get an update??? .. should be a two inserts… whats is wrong???
I have entities with one to one relation made like at the aexample, but It works ok, but generate a lot of queries when I do a select. At this link I have the problem. http://stackoverflow.com/questions/26660956/one-simple-select-takes-a-lot-of-time-using-hibernate
HI,
Please help me for below situation.
I have tables like:
CREATE TABLE “OTHER_ACTIVITY_DETAIL”
( “SNO” NUMBER NOT NULL ENABLE,
“OA_DEVELOPER” NUMBER NOT NULL ENABLE,
“OA_DATE” DATE,
“OA_TYPE” VARCHAR2(2) NOT NULL ENABLE,
“OA_EFFORT” NUMBER,
“OA_REMARKS” VARCHAR2(200),
“OA_PREDEFINE” VARCHAR2(4),
“ACCOUNT_CODE” VARCHAR2(5) NOT NULL ENABLE,
CONSTRAINT “OTHER_ACTIVITY_DTL_PK” PRIMARY KEY (“SNO”) ENABLE
)
** Note: OA_PREDEFINE may null in my situation.
CREATE TABLE “PRE_DEFINE_ACTIVITY”
( “SNO” NUMBER NOT NULL ENABLE,
“PRE_DEFINE_CODE” VARCHAR2(4) NOT NULL ENABLE,
“PRE_DEFINE_DESC” VARCHAR2(50),
“PRE_DEFINE_MONTH” DATE,
“ACCOUNT_CODE” VARCHAR2(5) NOT NULL ENABLE,
CONSTRAINT “PRE_DEFINE_ACTIVITY_PK” PRIMARY KEY (“SNO”) ENABLE
)
I used in hbm like :
Please help me to prepare HQL.
I am using Spring 4+ Hibernate 4 please suggest me how to achieve in XML mapping way.
Thanks,
Venkat.
Thanks for the awesome example!
getting following error:
java.lang.NullPointerException
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.tuple.entity.AbstractEntityTuplizer.getPropertyValue(AbstractEntityTuplizer.java:521)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.persister.entity.AbstractEntityPersister.getPropertyValue(AbstractEntityPersister.java:3842)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.id.ForeignGenerator.generate(ForeignGenerator.java:100)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.event.def.AbstractSaveEventListener.saveWithGeneratedId(AbstractSaveEventListener.java:121)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.event.def.DefaultMergeEventListener.saveTransientEntity(DefaultMergeEventListener.java:415)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.event.def.DefaultMergeEventListener.mergeTransientEntity(DefaultMergeEventListener.java:341)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.event.def.DefaultMergeEventListener.entityIsTransient(DefaultMergeEventListener.java:303)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.event.def.DefaultMergeEventListener.onMerge(DefaultMergeEventListener.java:258)
[9/20/13 18:14:38:411 IST] 0000002f SystemErr R at org.hibernate.impl.SessionImpl.fireMerge(SessionImpl.java:877)
Please also explain annotations it is difficult to understand without it.
please can someone specifically tell which hibernate jar files are used because i am getting following error
INFO: Configuration resource: /hibernate.cfg.xml
Initial SessionFactory creation failed.org.hibernate.MappingException: An AnnotationConfiguration instance is required to use
Exception in thread “main” java.lang.ExceptionInInitializerError
at util.HibernateUtil.buildSessionFactory(HibernateUtil.java:20)
at util.HibernateUtil.(HibernateUtil.java:9)
at mainclass.App.main(App.java:14)
Caused by: org.hibernate.MappingException: An AnnotationConfiguration instance is required to use
at org.hibernate.cfg.Configuration.parseMappingElement(Configuration.java:1692)
at org.hibernate.cfg.Configuration.parseSessionFactory(Configuration.java:1647)
at org.hibernate.cfg.Configuration.doConfigure(Configuration.java:1626)
at org.hibernate.cfg.AnnotationConfiguration.doConfigure(AnnotationConfiguration.java:213)
at org.hibernate.cfg.AnnotationConfiguration.doConfigure(AnnotationConfiguration.java:46)
at org.hibernate.cfg.Configuration.doConfigure(Configuration.java:1600)
at org.hibernate.cfg.AnnotationConfiguration.doConfigure(AnnotationConfiguration.java:201)
at org.hibernate.cfg.AnnotationConfiguration.doConfigure(AnnotationConfiguration.java:46)
at org.hibernate.cfg.Configuration.configure(Configuration.java:1520)
at org.hibernate.cfg.AnnotationConfiguration.configure(AnnotationConfiguration.java:183)
at org.hibernate.cfg.AnnotationConfiguration.configure(AnnotationConfiguration.java:46)
at org.hibernate.cfg.Configuration.configure(Configuration.java:1506)
at org.hibernate.cfg.AnnotationConfiguration.configure(AnnotationConfiguration.java:177)
at util.HibernateUtil.buildSessionFactory(HibernateUtil.java:14)
please do reply
Hello,
I have performance problem with that. see: http://stackoverflow.com/questions/16713114/performance-issue-with-primarykeyjoincolumn
Can you reply it?
Manu
I just use ur example in my project but data is not inserted
could u help me on this
Hi there! Do you know if they make any plugins to safeguard against hackers? I’m kinda paranoid about losing everything I’ve worked hard on. Any tips?
Hi,
I have the same question as Usman Awan. If the class stock has a member stockdetail, why
does the class stockdetail need to have a stock member?
thank you,
ph.
Great Job Mykong:)
It is a very helpful post 🙂
Can you do a one to one mapping between a Table and a View?
Great Job Mykong, i have tested the same code with hibernate 4.1,jpa 2,jboss eclipse plugin mysql, and it worked very well thank for sharing the same .
EntityManagerFactory enityMgrFactry;
EntityManager entityManager;
EntityTransaction entityTrans;
try
{
enityMgrFactry= Persistence.createEntityManagerFactory(“employeePersistenceUnit”);
entityManager = enityMgrFactry.createEntityManager();
entityManager.getTransaction().begin();
Stock stock = new Stock();
stock.setStockCode(“7052”);
stock.setStockName(“PADINI”);
StockDetail stockDetail = new StockDetail();
stockDetail.setCompName(“PADINI Holding Malaysia”);
stockDetail.setCompDesc(“one stop shopping”);
stockDetail.setRemark(“vinci vinci”);
stockDetail.setListedDate(new Date());
stock.setStockDetail(stockDetail);
stockDetail.setStock(stock);
entityManager.persist(stock);
entityManager.getTransaction().commit();
System.out.println(“Done”);
Hi,
The examples are so clear and much helpful.
I have a query on annotations, in case a junit test case method requires db call(instead of a db call i will be using csv files for fetching/inserting data for junits) along with @test, is @transactional required or not.
Regards,
Sarath
There are two ways to do one to one mapping in the Hibernate Using the annotation
1. With Intermediate table.
2. Without Intermediate table.
For detail information see the below link
http://javaworldwide.blogspot.in/2012/05/one-to-many-relationship-in-hibernate.html
its for One to Many
and not for One to One as u said.
plz check your link
Hi Mkyong,
It is a very helpful post , Just have one question:
Why did you make a property of Stock in StockDetail class, and upon saving you also did this:
stock.setStockDetail(stockDetail); // this is ok for me that stock contain stockdetial
stockDetail.setStock(stock); // now this is the question why stockDetail must have stock object?
Waiting for your reply.
Regards,
Usman
Sorry for the double post ! cause it cut off some words …
Hi,
First i want to thank you for all your great posts !
I have one question :
I am wondering if it is enough to only add unique = true without using UniqueConstraint annotation …
If you do so and if you add hibernate.hbm2ddl.auto (value:update), then hibernate creates for that field more than once ‘UNIQUE KEY’ !
Best Regards,
Atif