Hibernate component mapping example
Hibernate component represents as a group of values or properties, not entity (table). See following tutorial to understand how component works in Hibernate.
1. Customer Table
See below customer table.
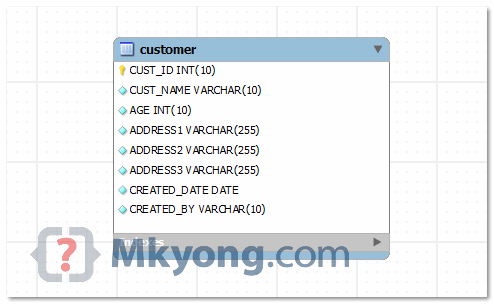
Customer table, SQL script in MySQL database.
CREATE TABLE `customer` (
`CUST_ID` int(10) unsigned NOT NULL AUTO_INCREMENT,
`CUST_NAME` varchar(10) NOT NULL,
`AGE` int(10) unsigned NOT NULL,
`ADDRESS1` varchar(255) NOT NULL,
`ADDRESS2` varchar(255) NOT NULL,
`ADDRESS3` varchar(255) NOT NULL,
`CREATED_DATE` date NOT NULL,
`CREATED_BY` varchar(10) NOT NULL,
PRIMARY KEY (`CUST_ID`) USING BTREE
);
2. Model Class
Now, create two model classes, Customer.java
and Address.java
, to represent the “customer” table above.
File : Customer.java
package com.mkyong.customer;
import java.util.Date;
public class Customer implements java.io.Serializable {
private Integer custId;
private String custName;
private int age;
private Address address;
private Date createdDate;
private String createdBy;
//setters and getters
}
File : Address.java
package com.mkyong.customer;
public class Address implements java.io.Serializable {
private String address1;
private String address2;
private String address3;
//setters and getters
}
In this case, Address.java
is a “component” represent the “Address1”, “Address2” and “Address3” columns for Customer.java
3. Component Mapping
Now, you can declares the component mapping like this :
File : Customer.hbm.xml
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.mkyong.customer.Customer" table="customer"
catalog="mkyongdb">
<id name="custId" type="java.lang.Integer">
<column name="CUST_ID" />
<generator class="identity" />
</id>
<property name="custName" type="string">
<column name="CUST_NAME" length="10" not-null="true" />
</property>
<property name="age" type="int">
<column name="AGE" not-null="true" />
</property>
<component name="Address" class="com.mkyong.customer.Address">
<property name="address1" type="string">
<column name="ADDRESS1" not-null="true" />
</property>
<property name="address2" type="string">
<column name="ADDRESS2" not-null="true" />
</property>
<property name="address3" type="string">
<column name="ADDRESS3" not-null="true" />
</property>
</component>
<property name="createdDate" type="date">
<column name="CREATED_DATE" length="10" not-null="true" />
</property>
<property name="createdBy" type="string">
<column name="CREATED_BY" length="10" not-null="true" />
</property>
</class>
</hibernate-mapping>
4. Run it
Create Address object, include it into the Customer object, and persist it.
package com.mkyong;
import java.util.Date;
import org.hibernate.Session;
import com.mkyong.customer.Address;
import com.mkyong.customer.Customer;
import com.mkyong.util.HibernateUtil;
public class App {
public static void main(String[] args) {
System.out.println("Hibernate component mapping");
Session session = HibernateUtil.getSessionFactory().openSession();
session.beginTransaction();
Address address = new Address();
address.setAddress1("Address 1");
address.setAddress2("Address 2");
address.setAddress3("Address 3");
Customer cust = new Customer();
cust.setCustName("mkyong");
cust.setAge(30);
cust.setAddress(address);
cust.setCreatedDate(new Date());
cust.setCreatedBy("system");
session.save(cust);
session.getTransaction().commit();
System.out.println("Done");
}
}
Output…
Hibernate component mapping
Hibernate:
insert
into
mkyongdb.customer
(CUST_NAME, AGE, ADDRESS1, ADDRESS2, ADDRESS3, CREATED_DATE, CREATED_BY)
values
(?, ?, ?, ?, ?, ?, ?)
Done
Download it – Hibernate-Component-Mapping-Example.zip (10KB)
Hi ,
I have written a mapping class where I want use “id” field of component class as my id in the main class. Can we do that ?
Thanks
Do you have to close / disconnect the Session??
how to do the mapping of component in JPA? eg in above example Address is not separate table in table then what is the table name we need to annote for Address Class.
thanks for this post
but i need sum help i have an error and i can’t remove it :
Exception in thread “main” java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
at org.hibernate.dialect.Dialect.(Dialect.java:58)
Caused by: java.lang.ClassNotFoundException: org.apache.commons.logging.LogFactory
at java.net.URLClassLoader$1.run(Unknown Source)
at java.net.URLClassLoader$1.run(Unknown Source)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
… 1 more
Is this obvious? java.lang.ClassNotFoundException: org.apache.commons.logging.LogFactory
Hi MkYong 🙂
Thanks for the Cool 🙂 Example 🙂
Sir could you please tell me why am i getting Duplicate values for the following code
MYSql Table ComboTable
My Pojo Hibernate Class
Hi MkYong 🙂
Thanks 🙂
i resolved it 🙂
it was my mistake i forgot to add
implements java.io.Serializable
Hi
Thanks for the post. Its really helpful. I have a question, why we go for component mapping instead we can declare the address properties in Customer class itself. Please clarify.
How about some tutorials about reading the information from a sql database using hibernate?